Hex to ASCII String Converter Online Tool
About Hex to ASCII String Converter Online Tool:
This online Hex to ASCII string converter tool helps you to convert one input Hex string (base 16) into a ASCII String.
The accept Hex string delimiters include ("", " ", "0x", "0X", "\0x", "\0X", "\x", "\X", "\n", "\t")
.
Hex Numeral System:
Hex numeral system (also called hexadecimal) has 16 digits, include (0, 1, 2, 3, 4, 5, 6, 7, 8, 9)
just like decimal numeral system, plus (a, b, e, d, e, f)
6 additional characters. Which
means it's is a number system of base 16. Since Hex numeral system can represent any binary string in a readable way, It is widely used in computer science. The SHA256 hash string is often appears as Hex style
string, the Color System used in HTML is also be written down as Hex number, from #000000
(pure black) to #FFFFFF
(pure white).
ASCII Encoding Standard:
ASCII (American Standard Code for Information Interchange) is the most widely used character encoding standard. The standard ASCII has 7 bits, 128 distinguish characters. The extended ASCII has 8 bits, 256
distinguish characters. The Copyright Symbol ©
that you see everyday is in the extended ASCII list.
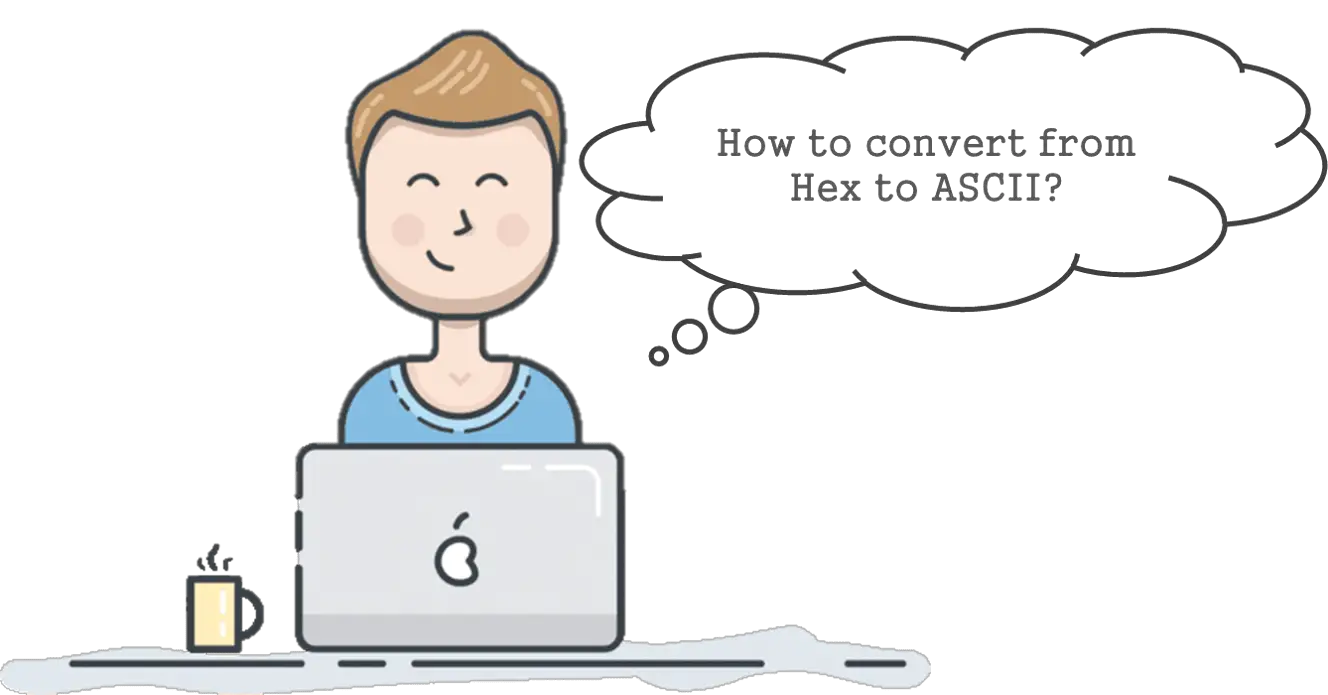
More information:
Wikipedia (Hexadecimal): https://en.wikipedia.org/wiki/Hexadecimal
Wikipedia (ASCII): https://en.wikipedia.org/wiki/ASCII
Convert Hex to ASCII String with Python:
import binascii def hex_to_ascii(hex_str): hex_str = hex_str.replace(' ', '').replace('0x', '').replace('\t', '').replace('\n', '') ascii_str = binascii.unhexlify(hex_str.encode()) return ascii_str hex_input = '54 68 69 73 20 69 73 20 61 6e 20 65 78 61 6d 70 6c 65 2e' ascii_output = hex_to_ascii(hex_input) print('ascii result is:{0}'.format(ascii_output)) ------------------- ascii result is:b'This is an example.'
Convert Hex to ASCII String with Java:
public class NumberConvertManager { public static String hex_to_ascii(String hex_str) { hex_str = hex_str.replace(" ", "").replace("0x", "").replace("\\x", ""); StringBuilder ascii_str = new StringBuilder(); for (int i = 0; i < hex_str.length(); i += 2) { String str = hex_str.substring(i, i + 2); ascii_str.append((char) Integer.parseInt(str, 16)); } return ascii_str.toString(); } public static void main(String[] args) { String hex_input = "54 68 69 73 20 69 73 20 61 6e 20 65 78 61 6d 70 6c 65 2e"; String ascii_output = hex_to_ascii(hex_input); System.out.println("ascii result is:" + ascii_output ); } } ------------------- ascii result is:This is an example.